Notice
Recent Posts
Recent Comments
Link
고양이 여름이의 지식채널
JAVA 파일 업로드, 다운로드 예제 본문
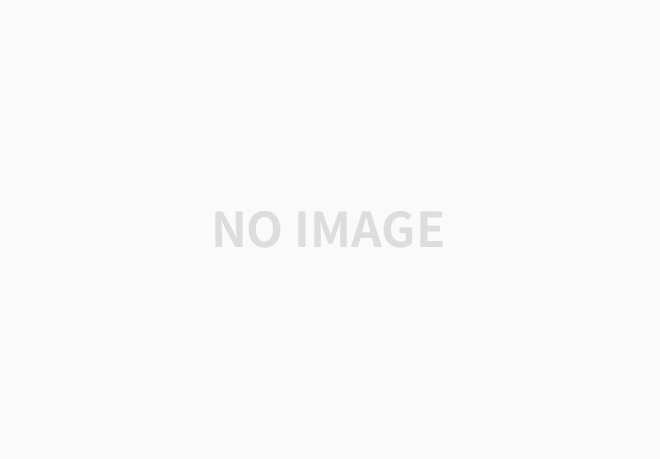
Java 파일 업로드와 다운로드 코드 예제입니다.
- io file copy
- FTP
- httpClient, multiparts
copy
io file 패키지의 copy 방식으로 파일 업로드, 다운로드 하는 코드 예제
Upload
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileUploadExample {
public static void main(String[] args) {
// 업로드 할 파일 위치
String sourceFilePath = "C:\\local\\directory\\file.txt";
// 업로드 위치
String destinationFilePath = "C:\\remote\\directory\\file.txt";
Path sourcePath = Paths.get(sourceFilePath);
try (InputStream inputStream = new FileInputStream(sourcePath.toFile())) {
Files.copy(inputStream, Paths.get(destinationFilePath));
} catch (IOException ex) {
System.out.println("Error: " + ex.getMessage());
ex.printStackTrace();
}
}
}
Download
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileDownloadExample {
public static void main(String[] args) {
// 다운로드 할 파일 위치
String sourceFilePath = "C:\\remote\\directory\\file.txt";
// 다운로드 받을 위치
String destinationFilePath = "C:\\local\\directory\\file.txt";
Path destinationPath = Paths.get(destinationFilePath);
try (OutputStream outputStream = new FileOutputStream(destinationPath.toFile())) {
Files.copy(Paths.get(sourceFilePath), outputStream);
} catch (IOException ex) {
System.out.println("Error: " + ex.getMessage());
ex.printStackTrace();
}
}
}
sourceFilePath 및 destinationFilePath 값을 업로드/다운로드할 파일에 적합한 값으로 바꿔서 사용하면 됩니다.
반응형
FTP
FTP패키지를 이용하여 FTP 서버로 파일 업로드, 다운로드 하는 코드 예제
Upload
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
public class FTPUploadExample {
public static void main(String[] args) {
//FTP 서버
String server = "ftp.example.com";
int port = 21;
String user = "username";
String password = "password";
//원격 파일 위치
String remoteFilePath = "/remote/directory/file.txt";
//로컬 파일 위치
String localFilePath = "C:\\local\\directory\\file.txt";
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
File localFile = new File(localFilePath);
FileInputStream inputStream = new FileInputStream(localFile);
ftpClient.storeFile(remoteFilePath, inputStream);
inputStream.close();
ftpClient.logout();
} catch (IOException ex) {
System.out.println("Error: " + ex.getMessage());
ex.printStackTrace();
} finally {
try {
if (ftpClient.isConnected()) {
ftpClient.disconnect();
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
Download
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
public class FTPDownloadExample {
public static void main(String[] args) {
//FTP 서버
String server = "ftp.example.com";
int port = 21;
String user = "username";
String password = "password";
//원격 파일 위치
String remoteFilePath = "/remote/directory/file.txt";
//로컬 파일 위치
String localFilePath = "C:\\local\\directory\\file.txt";
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
File localFile = new File(localFilePath);
FileOutputStream outputStream = new FileOutputStream(localFile);
ftpClient.retrieveFile(remoteFilePath, outputStream);
outputStream.close();
ftpClient.logout();
} catch (IOException ex) {
System.out.println("Error: " + ex.getMessage());
ex.printStackTrace();
} finally {
try {
if (ftpClient.isConnected()) {
ftpClient.disconnect();
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
서버, 포트, 사용자, 암호, remoteFilePath 및 localFilePath 값을 FTP 서버 및 업로드/다운로드할 파일에 적합한 값으로 바꿉니다.
Apache HttpClient
HttpClient 패키지를 이용하여 서버로 파일 업로드, 다운로드 하는 코드 예제
Upload
import java.io.File;
import java.io.IOException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
public class FileUploadExample {
public static void main(String[] args) throws IOException {
//파일 위치
String filePath = "C:\\local\\directory\\file.txt";
//업로드 URL
String uploadUrl = "http://example.com/upload";
File file = new File(filePath);
HttpEntity entity = MultipartEntityBuilder.create()
.addBinaryBody("file", file, ContentType.APPLICATION_OCTET_STREAM, file.getName())
.build();
HttpPost request = new HttpPost(uploadUrl);
request.setEntity(entity);
HttpClient httpClient = HttpClientBuilder.create().build();
HttpResponse response = httpClient.execute(request);
String responseBody = EntityUtils.toString(response.getEntity());
System.out.println(responseBody);
}
}
Download
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.HttpClientBuilder;
public class FileDownloadExample {
public static void main(String[] args) throws IOException {
//다운로드 파일 URL
String downloadUrl = "http://example.com/download/file.txt";
//다운로드 위치
String savePath = "C:\\local\\directory\\file.txt";
HttpGet request = new HttpGet(downloadUrl);
HttpClient httpClient = HttpClientBuilder.create().build();
HttpResponse response = httpClient.execute(request);
File file = new File(savePath);
FileOutputStream outputStream = new FileOutputStream(file);
response.getEntity().writeTo(outputStream);
outputStream.close();
}
}
filePath 및 uploadUrl의 값을 적합한 값으로 바꿔서 사용합니다.
728x90
반응형